22: C# Creating And Disposing Instances.
Take Up Code - Un pódcast de Take Up Code: build your own computer games, apps, and robotics with podcasts and live classes
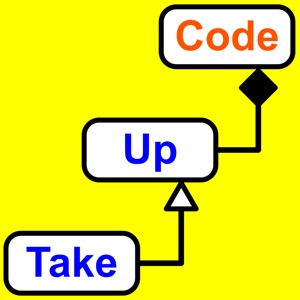
Categorías:
Creating instances in C# is a bit different because there’s a difference between value types and reference types. You’ll be able to navigate your objects with ease after this episode. You first learn how to place your C# classes in their own .cs files and how this is different than creating separate .cpp and .h files as in C++. You also have the ability in C# to declare your classes, structs, and even methods to be partial which allows you to divide them between multiple files. Then, you learn how to determine which types are value types and which are reference types. This is a new concept that at first seems similar to references in C++ but it’s actually a very fundamental difference in C#. Value type variables in methods always go to the stack directly and get their own instance. So if you have two instances of a struct type and you assign one of the variables to the other, what you end up doing is copying the contents of the one to the other. But a reference type just gives you a pointer that lives on the stack which actually points to the instance in the heap. C# doesn’t call this a pointer and it ends up looking very much like a reference in C++. If you create two instances of a class type and assign one of the variables to the other, what you end up with is both variables now point to the same instance. The values of the data inside the instance did not change and in fact, one of the instances will now be eligible for garbage collection. That’s all there is really to deleting instances in C#, you just forget about them and the garbage collector will clean it up at some later point. You can always implement Disposable and call the Dispose method if you have any action you need to perform before then. Just make sure to call Dispose while your reference still refers to the instance. Listen to the full episode or read the full transcript below for more. Transcript C# puts everything into source files called cs files and you normally create a cs file named after your class, struct, or interface that’s defined by that file. So if you have a class called Hero, then you would have just a Hero.cs file that defines the Hero class. If this was C++, then you would have separate Hero.h and Hero.cpp files. C# needs everything in cs files because the managed nature of the language relies on the runtime having access to everything. There’s no need to keep the implementation in a separate file. You can actually have a class split between multiple cs files in C# and this is really useful if, say, part of your class definition is generated by some automated tool while you write the rest. You don’t want your hand-written code getting overwritten when the tool creates an update. But the main thing is that no matter how many cs files are used to make a class definition, the runtime needs access to all of them. C# also supports structs which in C++ are almost identical to classes but are quite different in C#. Other than some limitations placed on structs, the biggest difference between the two in C# is that structs are value types while classes are reference types. You’ll see this difference when you create instance variables of your class or struct types. Before explaining the differences though, I want to mention that all the types in C# are either value or reference types. You’ll get a feel for which are which after a while but here’s the breakdown: Value types consist of structs, chars, bools, dates, enumerations, and all the numeric types. Reference types consist of classes, arrays, strings, and delegates. I know, we haven’t discussed all of these types. I’m trying to keep this episode focused on just what you need to know to be able to understand what happens when you create and dispose instances. Knowing that there are two main classifications of types that behave differently is enough for now. When you declare a variable in a method and give it a type and a