33: C++ Casting. Turn Your Object Into A Frog.
Take Up Code - Un pódcast de Take Up Code: build your own computer games, apps, and robotics with podcasts and live classes
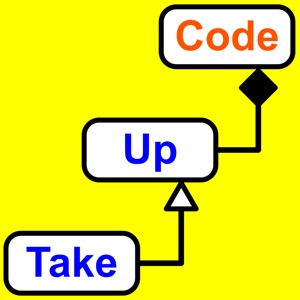
Categorías:
Can you change an int into a float? And because an int occupies multiple bytes in memory, can you get access to the individual bytes by themselves? Or what if you have a derived class and you want to refer to it as if it was a base class? And can you go the other way, from base class to derived class? These are the topics I’ll be explaining today. If you want to use an initializer list to construct an object, you can use it like this: int health {9.3}; instead of the older way that truncates: int health = 9.3; The C-style cast that I recommend you avoid looks like this to cast a long variable to an int variable: long count = 100; int shorterCount = (int)count; And there is the similar function style cast that I also recommend you avoid. It looks almost the same: long count = 100; int shorterCount = int(count); Instead of using a C-style or function style cast, you should instead use either an initializer list or one of the following named casts: const_cast static_cast reinterpret_cast dynamic_cast Listen to the full episode or read the full transcript below. Transcript There’s quite a bit of difference between casting in C++ and C# so today’s episode will focus on C++. The compiler will perform conversions for you when it needs to. Some of these are good and expected. Others will leave you wondering, “What were they thinking?” You can also step in and perform your own conversions and this is called casting. First, let’s take a simple example where you declare a new int variable called health and give it the initial value of 9.3. Your int variable can’t hold 9.3 so the compiler truncates the 9.3 value and your health variable ends up with just the value 9. If you want to prevent this automatic conversion from a floating point value to an integer value then instead of writing int health = 9.3, you can put the 9.3 in curly braces and then the equals sign becomes optional. You can just write int health, curly brace, 9.3, curly brace. This is called an initializer list and is a really nice and safe way to initialize your variables, including those that require multiple values. Converting from 9.3 to 9 is a narrowing conversion and loses some of the information. It’s sort of like what happens when you try to squeeze through a narrow opening and lose some skin along the way. If you’re worried about this happening to you, then you can always create a method that performs the assignment and then compares the result of the assignment back to the original value. If the values don’t match, then you lost some skin. Or you can use the curly brace initializer list which prevents this. The downside of the curly brace initializer list is that some conversions that might have made it through without being narrowed will now cause an error. Sometimes conversions will lose precision such as when converting from an integer to a float. You should realize that floats especially and even doubles can’t exactly represent all values. Have you ever tried writing out the decimal value of one third? It just keeps going right? 0.33333 etc. Other number systems such as used by computers have problems too. For instance, while we can write one tenth as 0.1 and one hundredth as 0.01, these simple fractions cause problems for computer floating point values just like one third does in decimal. If you’re writing a banking application, you might want to consider a special class to keep track of currency. Otherwise, you might find that your program has problems counting one cent pennies. This is not a problem with C++ or even C#. It’s just a matter of being aware of how computers represent and work with numbers. Another type of conversion that the compiler will do for you is called a promotion and this will not lose information. If you have a char value and assign that to an int, then it’s guaranteed to fit in either an int or an unsigned int. But the compiler will also promote bools